I like lambda-expressions. They have an elegance to them which, when I put into my code along with comments using the term "iff", probably marks me out as a Computer Scientist; the way people who studied Latin drop random phrases into sentences to communicate more precisely with others who did the same. Here, rather than use phases like "sue generis", I can drop in obscure references to Church's work, allude to "The Halting Problem" and say "tuple" whenever "pair" wouldn't be elitist enough.
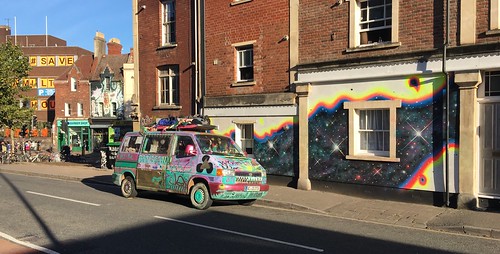
Really though, lambda-expressions are nice because they are a way to pass around snippets of code to use elsewhere
I've mostly used this in tests, with
LambaTestUtils.intercept()
being the code we've built up to use them, something clearly based on ScalaTest's work of the same name.protected void verifyRestrictedPermissions(final S3AFileSystem delegatedFS) throws Exception { intercept(AccessDeniedException.class, () -> readLandsat(delegatedFS)); }
I'm also working on wiring up the
UserGroupInformation.doAs()
call to l-expressions, so we don't have to faff around creating over-complex PrivilegedAction
subclasses, instead go bobUser.do(() -> fs.getUsername())
. I've not done that yet, but have the stuff in my tests to explore it: doAs(bobUser, () -> fs.getUsername())
.Java-8 has embraced this, with its streams API,
Optional
class, etc. I should be able to do the same elegant code in Java 8 that you can do in Scala, such as on an Optional<UserGroupInformation>;
instance —no more need to worry about null pointers!Optional<Credentials> maybeCreds = maybeBobUser.map.doAs( (b) -> b.getCredentials())
And I can the same on those credentals
List<TokenIdentifier> ids = maybeCreds.map(::getAllTokens).stream() .map(::decodeTokenIdentifier) .getOrElse(new LinkedList<>()).stream()
Except, well, I can't. Because of checked exceptions. That,
Token::decodeTokenIdentifier
method can raise IOException
instances whenever there's a problem decoding the byte array which contains the token identifier (it can also return null for other issues; see HADOOP-15808).All Hadoop API calls which do some kind of network or IO operation declare they throw an
IOException
when things fail. It's consistent, it works fairly well. Sometimes interactions with underlying libraries (AWS SDK, Azure SDK) we catch & map, but we also do other error translation there too, then feed that into retry logic and things even out. When you call getFileStatus()
against s3a: or abfs:// you can be confident that if its not there you'll get a FileNotFoundException
; if there was some connectivity issue our code will have retried, provided it wasn't something unrecoverable like a DNS/Routing problem, where you'll get a NoRouteToHostExcepotion
in your stack traces.Checked exceptions are everywhere in the Hadoop code.
And the Java Streams API can't work with that. All the operations on a stream don't declare that they raise exceptions, so none of the lambda-expressions you can call on them may either. I could jump through hoops and catch & convert them into some
RuntimeException
—but then what? All the code which is calling mine expects failures to come as IOExceptions
, expect those FileNotFoundExceptions
, etc. We cannot make serious use of the new APIs in our codebase.Now, the Oracle team could just have declared that the new
map()
method raised Exception
or similar, but then it'd have been unusable in those methods which don't declare that they throw exceptions, or those which say, throw IOExceptions. There's no obvious solution to this with those standard Java classes, leaving me the options of (a) not using them or (b) writing my own -which something I've been doing in places. I shouldn't have to do that, all it does is create maintenance pain and doesn't glue together with those standard libraries.
I don't have a choice. And neither does anyone else using Java. Scala doesn't have this problem as exceptions aren't checked. Groovy doesn't have this problem as exceptions aren't checked. C# doesn't have this problem as exceptions aren't checked. Java, however, is now trapped by some design decisions made twenty+ years ago which seemed a good idea at the time.
Is there anything Oracle can do now? I don't know. You could change the compiler to say "all exceptions are unchecked" and see what happens. I suspect a lot of code will break. And because it'll be on the failure paths where problems surface, it'd be hard to get that test coverage to be sure that failures are handled properly. Even so, I can imagine that happening, otherwise, even as the language tries to "stay modern", it's crippled.
No comments:
Post a Comment
Comments are usually moderated -sorry.